Introduction
Debouncing is a crucial technique in web development, especially when dealing with user input like search bars. In this tutorial, we’ll explore how to implement debouncing in Angular using ngModel for two-way data binding and RXJS for handling asynchronous operations.
Step 1: Create a new Angular Project
If you haven’t already, open your terminal and run the following commands:
ng new angular-debounce-tutorial
cd angular-debounce-tutorial
Step 2: Install RXJS
In your project directory, install RXJS:
npm install rxjs
Step 3: Import FormsModule and ReactiveFormsModule
Open src/app/app.module.ts
and make sure you import FormsModule
and ReactiveFormsModule
from @angular/forms
:
// app.module.ts
import { FormsModule, ReactiveFormsModule } from '@angular/forms';
@NgModule({
// ...
imports: [
// ...
FormsModule,
ReactiveFormsModule,
],
// ...
})
export class AppModule { }
Step 4: Create a Search Component
Generate a new component:
ng generate component search
Step 5: Implement Debouncing in the Search Component
Open src/app/search/search.component.ts
and modify it as follows:
// search.component.ts
import { Component } from '@angular/core';
import { Subject } from 'rxjs';
import { debounceTime, distinctUntilChanged } from 'rxjs/operators';
@Component({
selector: 'app-search',
templateUrl: './search.component.html',
styleUrls: ['./search.component.css']
})
export class SearchComponent {
searchTerm$ = new Subject<string>();
searchValue = '';
constructor() {
this.searchTerm$
.pipe(
debounceTime(300),
distinctUntilChanged()
)
.subscribe(searchTerm => this.handleSearch(searchTerm));
}
handleSearch(searchTerm: string) {
// Perform the search operation here
console.log(`Searching for: ${searchTerm}`);
this.searchValue = searchTerm;
// Can use the search value anywhere
}
}
Step 6: Update the Search Component Template
Open src/app/search/search.component.html
and modify it as follows:
<!-- search.component.html -->
<input [(ngModel)]="searchValue" (ngModelChange)="this.searchTerm$.next($event)" type="text" placeholder="Search...">
Step 7: Use the Search Component in App Component
Open src/app/app.component.html
and include the <app-search></app-search>
tag:
<!-- app.component.html -->
<div style="text-align:center">
<h1>
Welcome to {{ title }}!
</h1>
</div>
<app-search></app-search>
Step 8: Run Your Angular App
In your terminal, run:
ng serve
Open your browser and go to http://localhost:4200/
. You should see your Angular app with a search input.
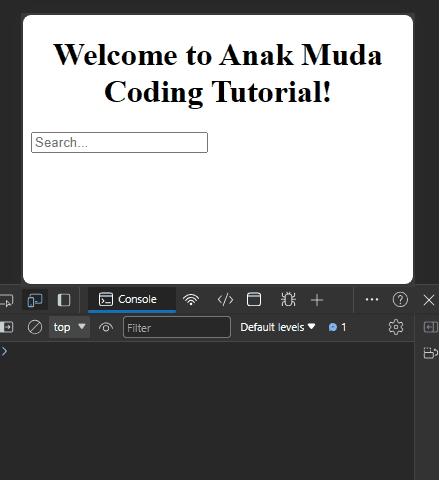
Conclusion:
Congratulations! You’ve successfully implemented debouncing in Angular using ngModel and RXJS. The debouncing technique enhances user experience by preventing unnecessary function calls during rapid input.
Feel free to experiment with different debounce times and explore additional RXJS operators to customize the behavior based on your application’s needs.
Learn more about Angular tips with us here.