Introduction
Welcome to this Angular tutorial! In this guide, we will explore how to dynamically render lists using Angular’s powerful ngFor
directive in combination with array slices. This technique allows you to selectively display a subset of items from an array, offering flexibility and control over your application’s user interface.
Step 1: Create a New Angular Project
Open your terminal and run the following commands:
ng new ngfor-slice-tutorial
cd ngfor-slice-tutorial
Step 2: Create a Component Named array-slice
Create a new component named array-slice
:
ng generate component array-slice
Step 3: Update the array-slice
Component
Open src/app/array-slice/array-slice.component.ts
and modify it to use ngFor
with array slices:
// src/app/array-slice/array-slice.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-array-slice',
templateUrl: './array-slice.component.html',
styleUrls: ['./array-slice.component.css']
})
export class ArraySliceComponent {
items = ['Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 5'];
startIndex = 1; // Adjust as needed
endIndex = 4; // Adjust as needed
}
Step 4: Update the array-slice
Component HTML File
Open src/app/array-slice/array-slice.component.html
and use ngFor
with array slices:
<!-- src/app/array-slice/array-slice.component.html -->
<h2>List of Items</h2>
<div *ngFor="let item of items.slice(startIndex, endIndex)">
{{ item }}
</div>
Step 5: Use the ArraySliceComponent
in the app
Component
Open src/app/app.component.html
and include the ArraySliceComponent
:
<!-- src/app/app.component.html -->
<div style="text-align:center">
<h1>
Welcome to {{ title }}!
</h1>
<app-array-slice></app-array-slice>
</div>
Step 6: Run the Application
In your terminal, run the following command:
ng serve
Open your browser and navigate to http://localhost:4200/
. You should see a list of items displayed based on the slice defined in the ArraySliceComponent
.
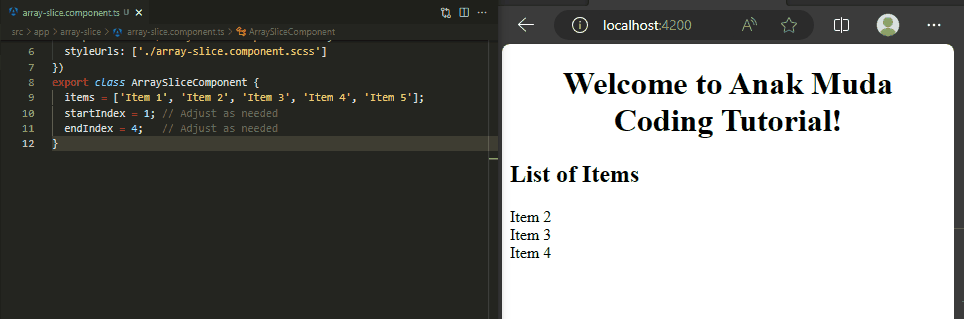
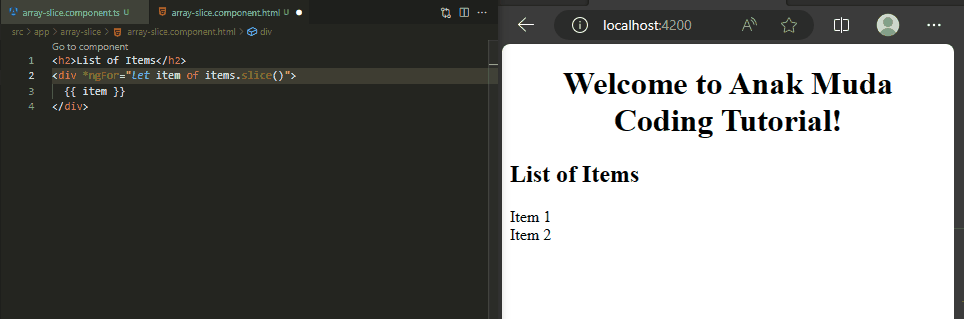
Conclusion
Congratulations! In this tutorial, we’ve explored how to use the ngFor
directive in Angular to dynamically render lists. By incorporating array slices, you can easily control which subset of items is displayed. This technique is valuable for creating responsive and user-friendly Angular applications.
Feel free to experiment further with different values for startIndex
and endIndex
or apply this knowledge to more complex scenarios in your Angular projects. Happy coding!
Learn more about Angular tips with us here.